Essay/Term paper: Fractable.cpp
Essay, term paper, research paper: High School Essays
Free essays available online are good but they will not follow the guidelines of your particular writing assignment. If you need a custom term paper on High School Essays: FracTable.cpp, you can hire a professional writer here to write you a high quality authentic essay. While free essays can be traced by Turnitin (plagiarism detection program), our custom written essays will pass any plagiarism test. Our writing service will save you time and grade.
//FracTable
#include <iostream>
#include <cmath>
#include <conio.h> //required for getch()
#include <iomanip> //required for setw()
using namespace std;
class fraction //fraction class
{
private:
int num,den;
public:
fraction(): num(0),den(0){}
fraction(int n,int d):num(n),den(d) {}
void display() {cout<<num<<"/";cout.setf(ios::left);cout<<setw(4)<<den;}
fraction mult(fraction&, fraction&);
void dispheader()
{cout <<num <<"/";cout.setf(ios::left);cout<<setw(4)<<den;}
void lowterms();
};
//----------------------Source copy from textbook----------------------------
void fraction::lowterms() // Change ourself to lowest terms
{
long tnum, tden, temp, gcd;
tnum = labs(num); // use non-negative copies
tden = labs(den); // (needs cmath)
if(tden == 0) // check for 0/n
{
cout << "Illegal fraction: division by 0"; exit(1);
}
else if(tnum==0) //check for 0/n
{
num = 0; den = 1; return;
}
//This 'while' loop finds the gcd of tnum tden
while(tnum != 0)
{
if(tnum < tden) // ensure numerator larger
{
temp = tnum; tnum = tden; tden = temp; //swam them
}
tnum = tnum - tden; // subtract them
}
gcd = tden; // this is greates comon divisor
num = num / gcd; // divide both num and den by gcd
den = den / gcd; // to reduce frac to lowest terms
}
//-----------------------End of source copy from textbook-------------------
int get_den() //get denominator from user
{
int valid =2;
int denom;
cout<< "Enter a denominator:a ";
cin>>denom; cout<<endl;
if (denom < valid)
do //loop until valid entry
{
cout<< "Invalid entry. Try again."<<endl;
cout<< "Enter a denominator:a " ;
cin>> denom; cout<<endl;
}
while(denom < valid);
return denom;
}
/*----------------------------------------------------------------*/
fraction fraction::mult(fraction& x,fraction& y)
{
fraction temp;
temp.num = x.num * y.num;
temp.den = x.den * y.den;
return temp;
}
/*----------------------------------------------------------------*/
int main()
{
int atemp,btemp,din;
int headmax(8);
int dashmax(7);
din=(get_den());
cout<<setw(headmax)<<" ";
for (atemp=1; atemp < din ; atemp++)
{
fraction x(atemp,din);
x.lowterms();
x.dispheader();
}
cout <<endl;
for (int temp = 1; temp <(din*dashmax); temp++)
{
cout<<"-";
}
for (atemp=1; atemp < din ; atemp++)
{
fraction x(atemp,din);
cout<<endl;
if (atemp < din)
{
x.lowterms();
x.display();
cout<<"| ";
}
for (btemp=1; btemp < din; btemp++)
{
fraction y(btemp,din);
fraction z= z.mult(x,y);
z.lowterms();
z.display();
}
}
cout<< endl<< "Press any key to terminate program.a";
getch(); //keep window open until key pressed
return 0;
}
Other sample model essays:
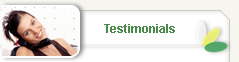

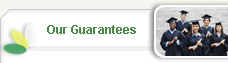

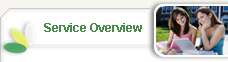

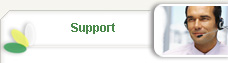

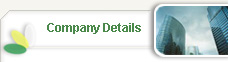
